Count up numbers with GSAP
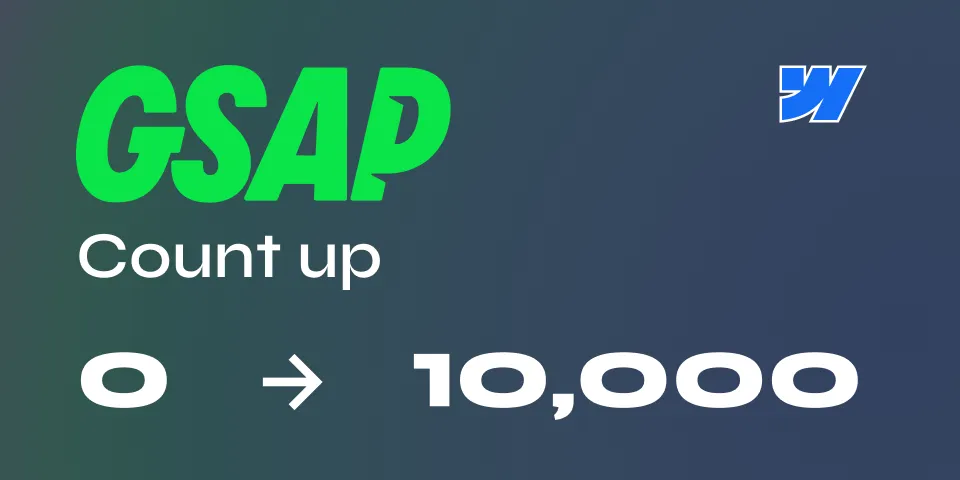
Count up numbers with GSAP
GSAP is well known for animating CSS properties in a performant way, but there is a trick to animate numbers (and other stuff, but let’s focus on numbers for now).
A very common use case is animating numbers from 0 to X, like a counter.
So a let’s say we want to showcase that we have worked on 200+ projects. Quite an achievement, right? 🎉
Write the number in the HTML, then make sure to add a span around the number 200 and give it a class/id/attribute.
We will then target that selector to animate it.
It will look something like this:
// get a reference to the elements we want to animateconst element = document.querySelector('.your-class')
gsap.from(element, { textContent: 0, // start from 0 duration: 2, // animate in 2 seconds snap: { textContent: 1 }, // increment by 1})
Boom! You have a counter that animates from 0 to 200 in 2 seconds.
If you want to use decimals, you can change the snap
value to 0.1, 0.01, etc.
You can even control the easing (check their easing visualizer for more info).
Format numbers
We can also format the numbers to have commas at the thousands, like 1,000, 10,000, etc.
We’ll use the onUpdate callback to format the numbers every time they change.
gsap.from(element, { textContent: 0, duration: 2, snap: { textContent: 1 }, onUpdate: function () { // on every increment, format the number element.textContent = parseInt(element.textContent).toLocaleString() },})
That’s nice already, right? 🎉
But as always, let’s take it a step further.
Animate numbers when they are in view
Let’s say we have a section with 3 numbers that we want to animate.
We just need to add a few more lines of code:
const triggerSection = document.querySelector('#section')const elements = document.querySelectorAll('.your-class')
gsap.from(elements, { textContent: 0, // start from 0 duration: 2, snap: { textContent: 1 }, // increment by 1 scrollTrigger: { trigger: triggerSection, // trigger section start: 'top center', // "start when the top of the element reaches the top of the screen" },})
(Note that you need to add the ScrollTrigger plugin for this to work)
And that’s it! You have a section with 3 numbers that will animate from 0 to their respective values in 2 seconds when the section is in view.
You can check out the demo I made in Webflow to see it in action.